IDS basic python script
Hi! Let me provide you with the steps to run and use this network intrusion detection script:
- Prerequisites:
- Ensure you have Python installed on your system. This script was written using Python 3.
- Install the Scapy library. You can do this by running the following command in your terminal or command prompt:
pip install scapy
- Download the script:
- You can either copy the code provided earlier in this conversation or download the script from a source code repository (e.g., GitHub).
- Save the script:
- Save the script to a directory of your choice, for example,
network_intrusion_detection.py
.
- Run the script:
- Open a terminal or command prompt and navigate to the directory where you saved the script.
- Run the script using the following command:
python network_intrusion_detection.py
- Observe the output:
- The script will start capturing and analyzing network packets in an infinite loop.
- If it detects any potential intrusion attempts, it will print the corresponding messages to the console.
- You can observe the output and monitor the number of processed packets.
- Stop the script:
- To stop the script, press Ctrl+C in the terminal or command prompt.
- The script will catch the
KeyboardInterrupt
exception and print a message indicating that it’s exiting.
That’s the basic process to run and use this network intrusion detection script. Here are a few additional notes:
- Make sure you have the necessary permissions to capture network traffic on your system. Depending on your operating system and network configuration, you may need to run the script with elevated privileges (e.g., as an administrator or with
sudo
on Unix-like systems). - This script is a simple proof-of-concept and does not provide a complete intrusion detection solution. In a production environment, you would need to integrate it with other security tools, log monitoring, and incident response processes.
- You can customize the script to monitor for additional types of attacks, adjust the detection criteria, or integrate it into a larger security monitoring framework.
from scapy.all import sniff, TCP, IP
import time
def detect_intrusion(packet):
"""
Analyzes a network packet and checks for signs of potential intrusion attempts.
Args:
packet (Packet): The network packet to be analyzed.
Returns:
None
"""
# Check if the packet contains TCP protocol
if TCP in packet:
# Check if the destination port is 22 (SSH) and the TCP flags indicate a SYN-ACK handshake
if packet[TCP].dport == 22 and packet[TCP].flags & 0x12 == 0x12:
# Detected a potential SSH brute-force attack
print("Possible SSH brute-force attack detected from", packet[IP].src, ":", packet[TCP].sport, "to", packet[IP].dst, ":", packet[TCP].dport)
# Check if the destination port is 3306 (MySQL) and the TCP flags indicate a SYN-ACK handshake
elif packet[TCP].dport == 3306 and packet[TCP].flags & 0x12 == 0x12:
# Detected a potential MySQL injection attack
print("Possible MySQL injection attack detected from", packet[IP].src, ":", packet[TCP].sport, "to", packet[IP].dst, ":", packet[TCP].dport)
else:
# No suspicious activity detected
print("No suspicious activity detected.")
def main():
"""
The main function that runs the network packet capture and intrusion detection.
Returns:
None
"""
packet_count = 0 # Initialize the packet counter
try:
# Run the packet capture and intrusion detection in an infinite loop
while True:
# Capture 100 packets and pass them to the detect_intrusion function
sniff(filter="ip", prn=detect_intrusion, count=100, store=False)
packet_count += 100 # Increment the packet counter
print(f"Processed {packet_count} packets.")
time.sleep(1) # Add a 1-second delay to prevent excessive CPU usage
except KeyboardInterrupt:
# Handle the KeyboardInterrupt exception when the user presses Ctrl+C
print("\nScript interrupted by user. Exiting...")
if __name__ == "__main__":
# Execute the main function if the script is run directly
main()
The purpose of this script is to monitor network traffic and detect potential security threats, such as SSH brute-force attacks and MySQL injection attacks.
Here’s a high-level overview of what the script does:
- It uses the Scapy library to capture and analyze network packets in real-time.
- The
detect_intrusion()
function is responsible for analyzing each captured packet and checking for specific patterns that could indicate an intrusion attempt.- It looks for TCP packets with destination ports 22 (SSH) or 3306 (MySQL) that have the SYN-ACK flag set, which could indicate a brute-force attack or SQL injection attempt.
- If a potential attack is detected, the function prints a message describing the type of attack and the source and destination details.
- If no suspicious activity is detected, the function simply prints a “No suspicious activity detected” message.
- The
main()
function is the entry point of the script. It runs in an infinite loop, continuously capturing network packets and passing them to thedetect_intrusion()
function for analysis.- It processes 100 packets at a time and keeps track of the total number of processed packets.
- After each batch of 100 packets, it prints the current packet count and adds a 1-second delay to prevent excessive CPU usage.
- The script also handles the
KeyboardInterrupt
exception, which is triggered when the user presses Ctrl+C to interrupt the script. When this happens, the script prints a message and exits.
In summary, this script is a basic network intrusion detection system that monitors network traffic and alerts the user to potential SSH brute-force attacks and MySQL injection attempts. It’s a starting point for implementing more advanced network security monitoring and incident response mechanisms.
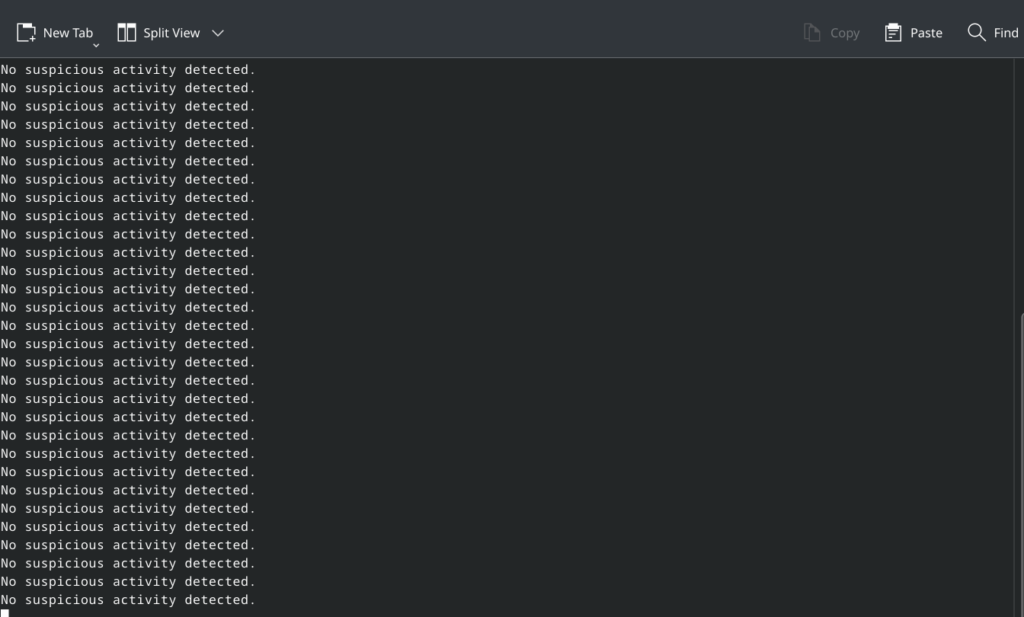